I recently found this image floating around on GamaSutra:
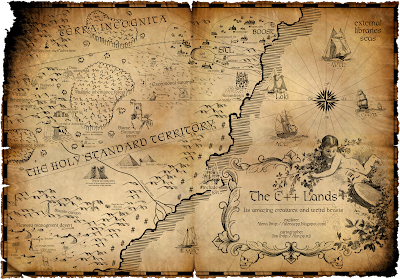
To the hopeless:
// The quick and easy way to set up an OpenGL perspective projection
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
glViewPort(0,0,640,480);
gluPerspective(60.0F, 1.33F, 0.01F, 500.0F); // 1.33 can be substituded for 1.337 if you're feeling particularly idiotic :3
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
// Render as normal
#include <cstdio>
#include <iostream>
#include <vector>
#include <string>
using namespace std;
vector<string> vec = {"This","is","one","of","many","new","features"};
class TClass
{
public:
TClass():number(0),data("Nothing"){}
TClass(int n, string d):number(n), data(d){}
~TClass(){}
string getdata(){return data;}
private:
int number;
string data;
};
// This is previously unheard of:
TClass g_tclass[] = {{0,"Pretty"},{1,"Darned"},{5,"Useful"}};
// How about this?
class CClass
{
public:
CClass(int x):x(x){}
CClass()
{
CClass(5); // Delegation
}
~CClass();
private:
int x;
}
// Here, have some more:
int main(int argc, char** argv)
{
auto var1 = "Auto types";
// Almost weak-typing, but not really, because
// adding in var1 = 4.55F; would be illegal right now.
// There is also declspec(), but it works differently.
// Both are determined at compile-time, meaning no speed impact.
// Lets print the data I defined earlier, the new way:
for(auto i : g_tclass)
cout << i.getname << endl;
// And the vector:
for(string i : vec)
cout << i << endl;
// Old method would be one of these two:
for(int i = 0; i < vec.size(); i++)
cout << vec[ i] << endl; // That was messing up my [ i] tags...
for(vector<string>::iterator i = vec.begin(); i != vec.end(); i++)
cout << (*i) << endl;
}
I like C++, it's not as complicated as C#. NET based is so confusing. I mean, c'mon,
I have no idea where the core of a Program is with C#, so tangled and it's like "wat".Although I do like C# so much more then a lot of languages.Just the C; Branches. :PI should point out that I would never, ever recommend actually interfacing with either DirectX or OpenGL using C++, ever, unless you want to develop your own engine instead of actually make a game. Use a library. If this is C# and XNA, fine. If your goal is to make a game, screw everyone else and just use whatever is easiest to use. There's a reason I'm developing language interfaces for my graphics engine - I believe you should be able to use whatever language you goddamn feel like using to make your game, and you should still be able to render 10000 images at the same time at 140 FPS. Performance concerns should be addressed at a low level, in graphics and physics and audio, not in the game logic.
A properly constructed game architecture will be able to annihilate the performance of almost all modern commercial games even if its using freaking python just by interfacing with well-optimized libraries. This includes using C-implemented data structures whenever possible and/or when appropriate. Game logic is so high-level performance almost entirely matters on the overarching layout of the program. So long as you pay attention to performance throughout development, you can write a highly efficient program in anything.Basically, in the simplest terms, as long as performance is given the attention it deserves, it's best to write a game (Or anything for that matter) in the language you're most comfortable in.
Though there definitely are cases where Python > C++, for instance.@Rob, What? This is my opinion. Not the 'literal truth', of how some people say it. Just because everyone else thinks C++ is the god-damn hardest language in the world doesn't mean it is. It all depends on the person learning the language.