I recently found this image floating around on GamaSutra:
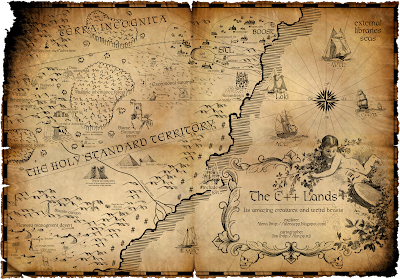
To the hopeless:
// The quick and easy way to set up an OpenGL perspective projection
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
glViewPort(0,0,640,480);
gluPerspective(60.0F, 1.33F, 0.01F, 500.0F); // 1.33 can be substituded for 1.337 if you're feeling particularly idiotic :3
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
// Render as normal
#include <cstdio>
#include <iostream>
#include <vector>
#include <string>
using namespace std;
vector<string> vec = {"This","is","one","of","many","new","features"};
class TClass
{
public:
TClass():number(0),data("Nothing"){}
TClass(int n, string d):number(n), data(d){}
~TClass(){}
string getdata(){return data;}
private:
int number;
string data;
};
// This is previously unheard of:
TClass g_tclass[] = {{0,"Pretty"},{1,"Darned"},{5,"Useful"}};
// How about this?
class CClass
{
public:
CClass(int x):x(x){}
CClass()
{
CClass(5); // Delegation
}
~CClass();
private:
int x;
}
// Here, have some more:
int main(int argc, char** argv)
{
auto var1 = "Auto types";
// Almost weak-typing, but not really, because
// adding in var1 = 4.55F; would be illegal right now.
// There is also declspec(), but it works differently.
// Both are determined at compile-time, meaning no speed impact.
// Lets print the data I defined earlier, the new way:
for(auto i : g_tclass)
cout << i.getname << endl;
// And the vector:
for(string i : vec)
cout << i << endl;
// Old method would be one of these two:
for(int i = 0; i < vec.size(); i++)
cout << vec[ i] << endl; // That was messing up my [ i] tags...
for(vector<string>::iterator i = vec.begin(); i != vec.end(); i++)
cout << (*i) << endl;
}
Hmn, the thumbnail tag seems to be a little slow on big images.
Yes, it does. Can't think of a way to fix it, unless it was server-side (Create a thumbnail cache).
Resize the image manually and upload to the file manager then use an image/url tag combo.
C++0x/11:
Great… but C++'s getting too complex. Scratch that. C++ is horribly complex, but I'm really use to it as it is, and find the idea of more complexity frightening. But it gets my +1 for having threads, hash maps, shared pointers. As much as the whole concept of 'Concepts' Makes Sense, I'm glad I don't have to worry about them, and I'm glad their not in the standard.There. Fixed it.
@ludamad: TBH, if you're going to even try use C++, you must be prepared for complexity. Just as an interesting potential scenario from life: You nab a job at some company or other, and a few weeks in you have to rewrite the firmware for an elevator control device. Then you're going to be using a struct ROM-compatible subset of the language, with complex inlines (Assembly, not inline methods). And C++ has been complex ever since they added in the concept of Multiple Inheritance. No, scratch that, ever since the language was born. But that's besides the point: Programming is complex.my god that is like the best thing
Mega: Oh believe me. I understand that C++ is complex. And that programming is complex. But that doesn't mean it should be arbitrarily complex, just because it already has X amount of complexity.
Also, 'auto' is nothing like weak typing. It's rudimentary type inference : ). boost::any on the other hand …There is a far bigger concern though I have with C++ than its complexity, believe me, I'm C++'s biggest advocate. It's the damn compile time. ConceptGCC, the preliminary implementation of Concepts, is known to be impossibly slow. (Of course, it would have got a lot better with time if Concepts were in the standard, but the point stands).The compile time isn't necessarily due to any specific feature, but due to the interaction of features such as conditional compilation, templates interacting with many other features and existing only in headers, etc, that cause much duplication of effort for the compiler. I would love a language that captures the beauty of C++ and richness of features in a much cleaner language. (In fact, I'm designing one, albeit much less focused on efficiency.)Also efficiency isn't everything. A language must first prove itself in usability, efficiency should always be an afterthought, once proven necessary.Anywho. I will continue to use C++ for years to come. And I will happily embrace the new standard. It is truly a unique language that you have to use for quite a while to appreciate.It boils down, as usual, to something I have to force myself to remember: Use the right tool for the job. I'd definitely not want to even think of any language but C++/C for creating a kernel, (Of course with some C++), but I'd rather use Python for anything involving creating a parser, Java for threading, either for networking, etc.
As for compilation time, it's often a moot point (With the exception of ConceptsGCC). You have to use design patterns to reduce dependencies, thus reducing compile time (Unless you rebuild your project).While -carefully- designed C++ can drastically reduce compile time, any rich use of C++ features such as highly nested templates etc will cause much overhead in terms of compile time. But yes it is an important tool as a C++ programmer. In my game I have one module that links to the main game that uses Boost out of necessity (for threading + networking), and the rest is blissfully unaware of any Boost headers.
And we mustn't forget the ability to copy+paste the definitions we need from header files if we're not using too many of them. I sometimes do that with the GLU library.