I recently found this image floating around on GamaSutra:
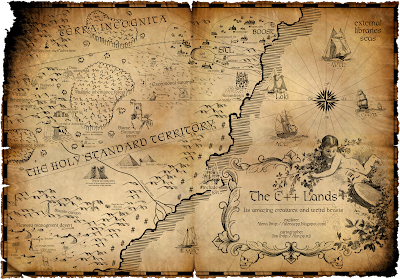
To the hopeless:
// The quick and easy way to set up an OpenGL perspective projection
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
glViewPort(0,0,640,480);
gluPerspective(60.0F, 1.33F, 0.01F, 500.0F); // 1.33 can be substituded for 1.337 if you're feeling particularly idiotic :3
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
// Render as normal
#include <cstdio>
#include <iostream>
#include <vector>
#include <string>
using namespace std;
vector<string> vec = {"This","is","one","of","many","new","features"};
class TClass
{
public:
TClass():number(0),data("Nothing"){}
TClass(int n, string d):number(n), data(d){}
~TClass(){}
string getdata(){return data;}
private:
int number;
string data;
};
// This is previously unheard of:
TClass g_tclass[] = {{0,"Pretty"},{1,"Darned"},{5,"Useful"}};
// How about this?
class CClass
{
public:
CClass(int x):x(x){}
CClass()
{
CClass(5); // Delegation
}
~CClass();
private:
int x;
}
// Here, have some more:
int main(int argc, char** argv)
{
auto var1 = "Auto types";
// Almost weak-typing, but not really, because
// adding in var1 = 4.55F; would be illegal right now.
// There is also declspec(), but it works differently.
// Both are determined at compile-time, meaning no speed impact.
// Lets print the data I defined earlier, the new way:
for(auto i : g_tclass)
cout << i.getname << endl;
// And the vector:
for(string i : vec)
cout << i << endl;
// Old method would be one of these two:
for(int i = 0; i < vec.size(); i++)
cout << vec[ i] << endl; // That was messing up my [ i] tags...
for(vector<string>::iterator i = vec.begin(); i != vec.end(); i++)
cout << (*i) << endl;
}
I use MSVC++ and I just set multi core compiling to "yes", it compiles medium sized projects in a few seconds with my i7.
As for the standard, it is long overdue as the popularity of boost shows.
1013Games: Multi-core compilation is the standard nowadays for sure. Still. My game takes a half minute to compile on 4 cores (from clean, anyway). And I have numerous compilation speeding techniques. Couldn't agree more @ boost though. I found using boost almost inescapable as my project grew, even though I didn't particularly want to use it.
Mega: That's a hackish technique that I really prefer to avoid.Also, another technique worth mentioning is using ccache. Helps with full rebuilds a lot / changing headers used frequently.Anyone who says that efficiency and speed should be an afterthought has apparently not noticed the fact that almost all modern software is horrendously slow and inefficient.
Gee, I wonder why that is.C++11 in the vast majority of cases is vastly superior to C++ in terms of speed, primarily due to move semantics and the massive benefits they bring. I'm sure you can find a way to abuse it into being slow, but that's never been particularly difficult with C++.The compilation time required for C++ is a serious problem. I would look forward to a redesign of the language that kept most of the features but implemented the syntax in a manner that is more easily parsed. D would be nice except it really likes garbage collection, thus destroying the entire point of using a low-level language. If you don't care about managing your memory manually, why bother using anything even resembling C++? Go use Haskell or some other vastly more elegant language.I avoid boost because of its abhorrent compile times. I use a series of precompiled headers to reduce compile times to something manageable and avoid using zillions of templates. If you are compiling a project in a few seconds, its not a complicated project. A complicated project on a full powered CPU takes about 10 seconds to compile from scratch.I find the fact that people think C++11 adds complexity amusing. At most it adds optional move semantics and new syntax for lambdas. C++11 auto, constructor improvements and variadic templates go a long way towards significantly reducing complexity. User-defined literals allow simplification of common operations. C++11 lets you write much higher-level, simplistic code, allowing C++ to be one of the few truly vertical languages capable of going from rudimentary functional programming all the way down to assembly.blackhole: Optimize after measuring a problem is what I meant. If the code doesn't work, it's useless. Slow code is still very useful depending on the problem. @Modern software being horrendously slow, that's a REALLY sweeping statement… And yes, when using boost in my game I was careful to link the parts of boost I needed to separate a library that I compile and then link to, making sure boost headers don't creep into my program.
"Significantly reducing complexity" this dubious at best. All the existing features will remain and even more ways of doing things continue to be piled on. Move constructors interacting with templates & exceptions anyone ?C++ programmers:
http://code.google.com/p/lanartsOpen source game I'm working on, help would be greeeatly appreciated.You think modern software being horrendously slow is a sweeping statement? I can prove it. They had to optimize the crap out of firefox to stop it from being bloated. Everything microsoft makes, save a few exceptions, is horrendously slow and bloated. Try opening word in less than 3 seconds. Turn on the pagefile in windows and watch it try to page out memory for no reason in a laughably incompetent manner. Visual studio is the most horrendously bloated program I've ever seen in my life. Even TortoiseSVN and TortoiseHg have badly designed workspaces and log viewers that lock up and lag terribly while attempting to load information. Almost all modern games are complete disasters. Skydrive and Google drive both suck up half your CPU trying to sync. Dropbox (programmed in python last I heard) does a much better job, which illustrates my point much better - You don't need to use C++ to make an efficient program. You just have to not disregard performance until the very end, because it's not something you can just slap on. You have to build the entire architecture to be performance-aware if you are going to optimize it later on. The overarching design choices of a program are what impact performance. It gets even worse with all the libraries I use. CEGUI is a bloated disaster that only manages to be fast in certain highly optimized cases, and the instant you step out of those it starts crashing down under the weight of its own horribly designed shit.
You want efficient software, you have to go to Linux, and then you can't use any of it because its GUI is shit. Java takes forever to load even the simplest programs because it can't load just simple programs, it loads up an entire virtual machine that can't properly garbage collect to save its life. Then there's Flash, and Photoshop, and everything Adobe makes, and then there's everything Apple makes. Eventually you end up either with efficient software that looks like shit and is difficult to use, or bloated pieces of crap that look pretty and are difficult to use for completely different reasons.I really fucking hate modern software development.Move constructors have nothing to do with exceptions. Move constructors usually add almost zero complexity to templates unless you want to forward an argument, in which case there's some special stuff you do for that. Even then, if you are worried about increased complexity in templates, you are looking at this from a library developer's point of view, not an application developer's point of view. If you are programming libraries, you have to use tons of complexity that is already present in C++ just to implement psuedo-versions of most of the new features in C++11 (functors, trying to optimize copy semantics so performance doesn't crash, etc.). Consequently, the "added" complexity involved takes away enough complexity that would otherwise be there that there is a net loss in complexity. It makes this stuff easier. If you disagree, then kindly explain to me how C++11 made it easier for me to write and maintain my various libraries, and how it made it VASTLY easier to then utilize these libraries in my applications.Net loss of complexity.I nearly missed out on this, and was about to disregard this blog in favor of a new one :3
Basically, I have to agree with blackhole on almost all the points (Except for the GUI. Some programs require a GUI, others are better suited to using a CLI, but in general, yes, the Linux GUI's are shit).So far, working with C++11 I've been finding the overall complexity and number-of-things-I-have-to-remember being reduced. But as usual, new standard and people are going to find 'anything' wrong with it, just for the sake of it.I'll just stick to C# for XNA and Unity. I've gotten depressed over both C++ and DirectX. If I take time to learn it again, I'll do it at my own pace without having to deal with the clusterfuck of a curriculum we have here at college.
Lost and alone, and looking for fucks to give.
C++, why you so picky?